What if I told you that creating empty objects can efficiently manage memory. But How? Well, at the starting point, you can initialize an empty object, and as the application progresses, you can add properties and values to the object, preventing memory leaks and not occupying unnecessary space.
To efficiently create an empty object in JavaScript, you can use the “Object literal ({ })” or “Object.create()” function. There is also a less efficient way called “Object constructor (new Object)”.
Method 1: Using Object literal
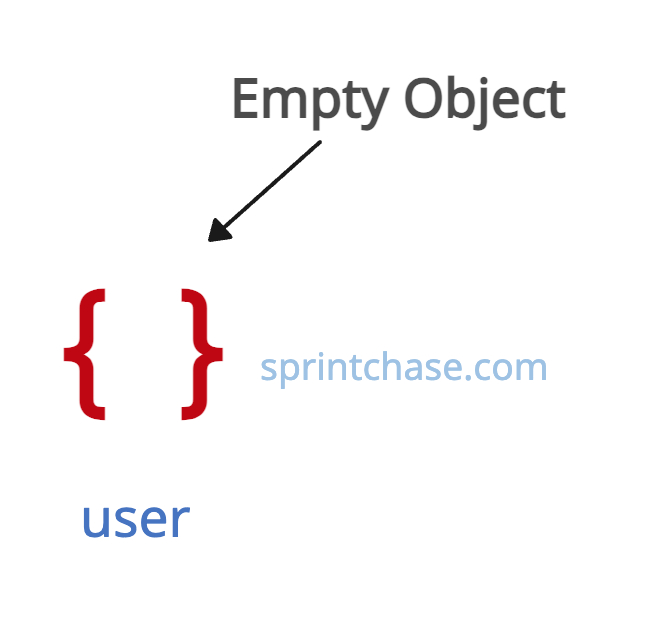
The object literal ({}) is the most efficient way and straightforward way. Assign the “{}” to a variable, and it becomes an empty object.
let user = {} console.log(empty_object) // {}
We now have our emptied user object. You can add as many properties as you want, which will increase the memory size.
let user = {} // Adding properties dynamically user.name = "Sagar"; user.age = 35; user.email = "sagar@sprintchase.com"; // Accessing and modifying properties console.log(user.name); // Output: Sagar user.age = 31; // Creating a nested object for address user.address = {}; user.address.street = "123 Main St"; user.address.city = "Anytown"; console.log(user) // Output: // { // name: 'Sagar', // age: 31, // email: 'sagar@sprintchase.com', // address: { street: '123 Main St', city: 'Anytown' } // }
Method 2: Using Object.create()

You can use the Object.create() method to create an empty object without a prototype by passing a “null” argument.
But you must have a big question. Why should anyone create an empty object without a prototype? The answer is simple: By using “null” as a prototype. The newly created object does not inherit properties from “Object.prototype”. This can be performance efficient if you are dealing with large objects.
const empty_object = Object.create(null); // Creates an object without a prototype console.log(empty_object) // [Object: null prototype] {}
Method 3: Using Object constructor (new Object())
The Object constructor is a less efficient way of constructing an empty object than the other two because it creates a new object instance.
const empty_object = new Object() console.log(empty_object) // {}That’s all!