Whether you want to prevent duplicate object entries from an array or avoid redundant operations, you must check if an array contains a specific object in JavaScript.
Objects are not primitive values and are compared by reference, not by value. Two objects with the same properties cannot be considered equal without having the same object instance.
In JavaScript, an array is a collection of values or objects. It provides different ways to access or check specific data when dealing with an array.
There are two efficient ways to check if an array includes an object:
- Using Array.includes()
- Using Array.some()
Method 1: Using Array.includes()
The array.includes() is a built-in method that verifies whether an array contains a specific value or object. Checking primitive values is easy compared to objects.
The array.includes() method is the most efficient one because it checks the existence of an object by reference. It only returns true if it finds a reference to an object; otherwise, it is false.
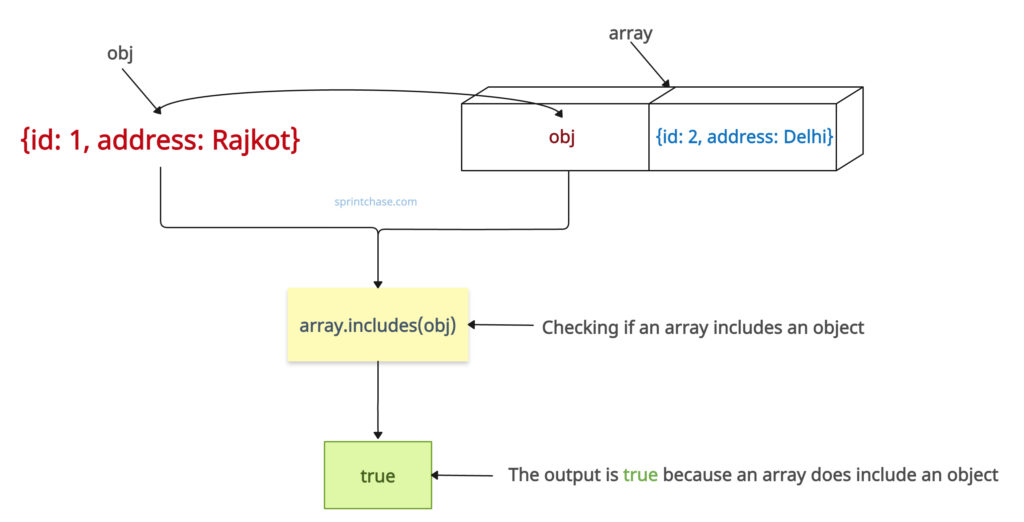
let obj = { "id": 1, "address": "Rajkot" } let array = [ obj, { "id": 2, "address": "Delhi" } ] let if_exists = array.includes(obj); console.log(if_exists) // Output: true
You can see that an array definitely contains an obj, and hence, .includes() returns true.
If you check for an object that does not exist in the array, it returns false.
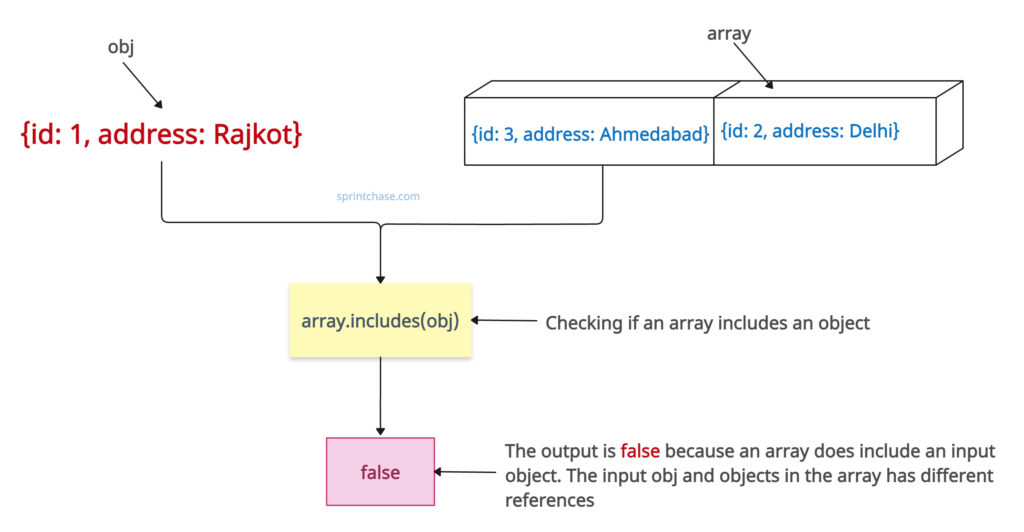
let obj = { "id": 1, "address": "Rajkot" } let array = [ { "id": 3, "address": "Ahmedabad" }, { "id": 2, "address": "Delhi" } ] let if_exists = array.includes(obj); console.log(if_exists) // Output: false
The array has two objects, but it does not match with the defined “obj”, and hence, it returns false.
In the above code example, even if your array contains “id”: 1 but “address” is different in the first object of the array, it will still return false.
let obj = { "id": 1, "address": "Rajkot" } let array = [ { "id": 1, "address": "Ahmedabad" }, { "id": 2, "address": "Delhi" } ] let if_exists = array.includes(obj); console.log(if_exists) // Output: false
The main advantage of the .includes() method is that it provides a concise and more readable syntax for checking whether an element exists and avoids unnecessary iterations over the entire array.
Method 2: Using Array.some()
Using array.some() method, you can check if an array includes an object by specific properties instead of checking an object by reference.
The array.some() function executes a custom function on each element of the array and checks each property of an object to see if it exists in the array. If it finds the exact object with exact properties, it returns true; otherwise, it is false.
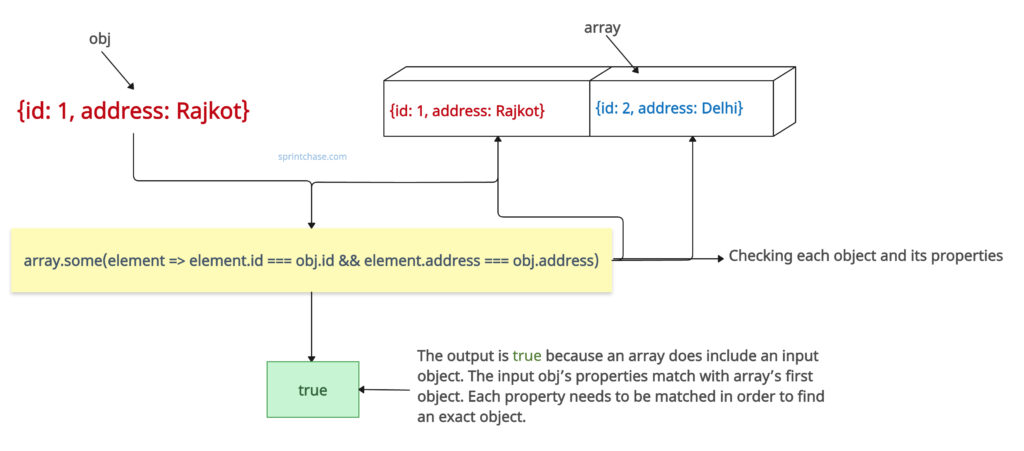
let obj = { "id": 1, "address": "Rajkot" } let array = [ { "id": 1, "address": "Rajkot" }, { "id": 2, "address": "Delhi" } ] let if_exists = array.some(element => element.id === obj.id && element.address === obj.address); console.log(if_exists) // Output: true
We are checking the “id” and “name” properties, and since we found a match, it returns true.
If any of the property’s values differ inside from the array’s first object, it will return false.
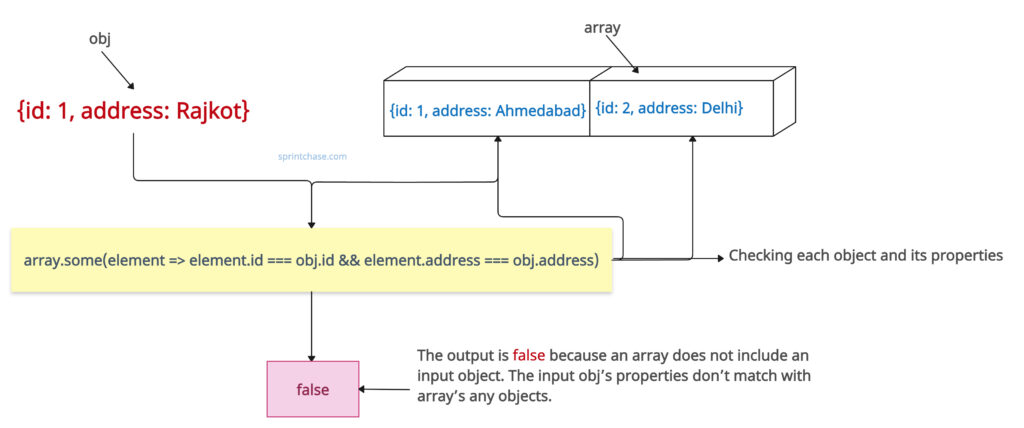
let obj = { "id": 1, "address": "Rajkot" } let array = [ { "id": 1, "address": "Ahmedabad" }, { "id": 2, "address": "Delhi" } ] let if_exists = array.some(element => element.id === obj.id && element.address === obj.address); console.log(if_exists) // Output: false
The array’s first object’s property “address” is “Ahmedabad”, which is different from obj’s “Rajkot.” Hence, it returns false.
When working with complex objects, you must implement a deep comparison to check for equality based on property values.